Published
- 6 min read
httpx - 3 powerfull automation tools
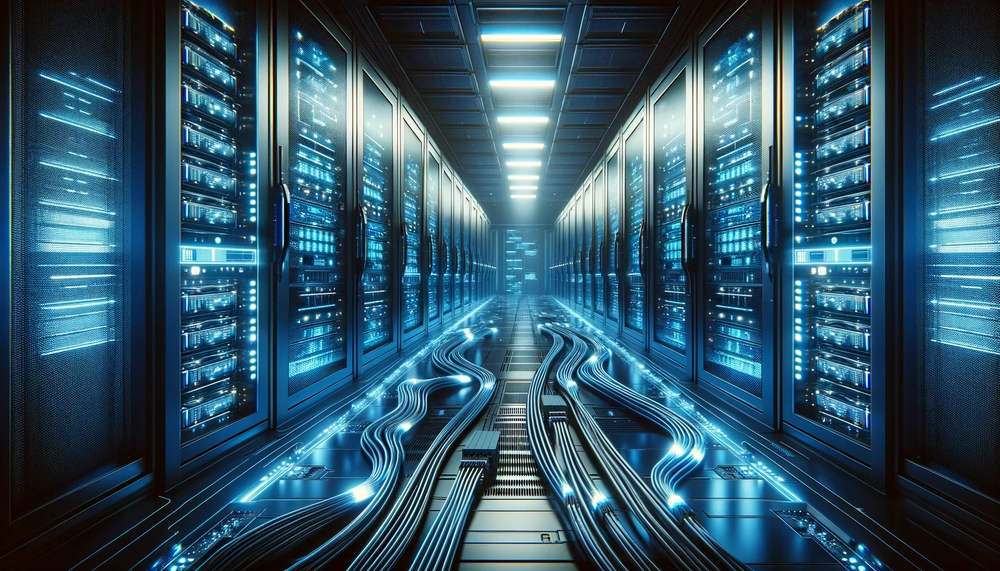
httpx x3
There’s 3 different httpx
tools:
- The bash tool
- The python cli tool
- The python library
Here’s how you can use them.
httpx - the fuzzing tool
$ httpx
__ __ __ _ __
/ /_ / /_/ /_____ | |/ /
/ __ \/ __/ __/ __ \| /
/ / / / /_/ /_/ /_/ / |
/_/ /_/\__/\__/ .___/_/|_|
/_/
projectdiscovery.io
[INF] Current httpx version v1.3.5 (latest)
Introduction
httpx is a fast web application reconnaissance
, automation
and enumeration
tool written in Go. It’s known for its speed and ease of use, making it a valuable tool for Bug Bounty Hunters and analysts.
Installation of go
These days, you can go with the repo version of go (>=1.18).
$ sudo apt install golang
Installation of httpx
$ go install -v github.com/projectdiscovery/httpx/cmd/httpx@latest
Basic Usage
httpx accepts STDIN input for scanning. Run a basic scan with options:
$ echo "http://vulnerable.com" | httpx
$ echo "http://vulnerable.com" | httpx -title -status-code -tech-detect -follow-redirects
Subdomain enum using subfinder and scan
Use subfinder to enumerate subdomains and feed them to httpx:
$ subfinder -d vulnerable.com | httpx -title -status-code -tech-detect -follow-redirects
Content probe
Use probes to refine scan results:
$ httpx -l list -path /robots.txt -sc
Content comparers
Use comparers to narrow down results:
$ cat list | httpx -mc 200,301,302 -sc
Content filters
Apply filters to eliminate results:
$ cat list | httpx -sc -fc 404
Rates and Timeouts
Control scan rates and timeouts:
$ cat list | httpx -sc -probe -t 10 -rl 1 -rlm 600
Show Responses and Requests
View HTTP requests and responses:
$ echo "http://vulnerable.com" | httpx -debug
Filtering for SQL Injections
Filter for SQL injection vulnerabilities:
$ echo "http://vulnerable.com" | httpx -path "/listproducts.php?cat=1’" -ms "Error: You have an error in your SQL syntax;"
Filtering for XSS reflections
Filter for reflected XSS vulnerabilities:
$ echo "http://vulnerable.com" | httpx -path "/listproducts.php?cat=<script>alert(1)</script>" -ms "<script>alert(1)</script>"
Web Page Fuzzing
Fuzz web pages using the -path module:
$ echo "http://vulnerable.com" | httpx -probe -sc -path "/login.php"
File output
Export scan results to files in different formats:
$ cat list | httpx -sc -o /root/results.txt
$ cat list | httpx -sc -csv -o /root/results.csv
$ cat list | httpx -sc -json -o /root/results.json
$ cat list | httpx -sc -o /root/results.txt -srd /root/responses
TCP/IP customizations
Customize TCP/IP filters for in-depth reconnaissance:
$ echo "http://network-sec.de" | httpx -pa -probe
$ echo "http://network-sec.de" | httpx -p 22,25,80,443,3306 -probe
POST Login
Send POST requests and log in:
$ echo "http://vulnerable.com" | httpx -debug-resp -x post -path "/userinfo.php" -H "Cookie: login=test%2Ftest" -body "uname=test&pass=test"
HTTP Methods Probe
Probe all HTTP OPTIONS (request methods):
$ echo "http://vulnerable.com" | httpx -x all -probe
Routing through proxy
Route HTTP requests through custom proxies:
$ echo "http://vulnerable.com" | httpx -x all -probe -http-proxy http://127.0.0.1:8080
httpx - the Python module cli tool
This is a completely different tool.
$ pip install httpx[cli,http2]
For sanity reasons, we renamed httpx
to httpx-cli
and will continue throughout the writeup.
$ httpx-cli --help
HTTPX 🦋
A next generation HTTP client.
Usage: httpx <URL> [OPTIONS]
-m, --method METHOD Request method, such as GET, POST, PUT, PATCH, DELETE, OPTIONS, HEAD.
[Default: GET, or POST if a request body is included]
-p, --params <NAME VALUE> ... Query parameters to include in the request URL.
-c, --content TEXT Byte content to include in the request body.
-d, --data <NAME VALUE> ... Form data to include in the request body.
-f, --files <NAME FILENAME> ... Form files to include in the request body.
-j, --json TEXT JSON data to include in the request body.
-h, --headers <NAME VALUE> ... Include additional HTTP headers in the request.
--cookies <NAME VALUE> ... Cookies to include in the request.
--auth <USER PASS> Username and password to include in the request. Specify '-' for the password to use a password prompt. Note
that using --verbose/-v will expose the Authorization header, including the password encoding in a trivially
reversible format.
--proxies URL Send the request via a proxy. Should be the URL giving the proxy address.
--timeout FLOAT Timeout value to use for network operations, such as establishing the connection, reading some data, etc...
[Default: 5.0]
--follow-redirects Automatically follow redirects.
--no-verify Disable SSL verification.
--http2 Send the request using HTTP/2, if the remote server supports it.
--download FILE Save the response content as a file, rather than displaying it.
-v, --verbose Verbose output. Show request as well as response.
--help Show this message and exit.
Sending a GET Request
$ httpx-cli https://jsonplaceholder.typicode.com/posts/1
Sending a POST Request with JSON Data
$ httpx-cli -m POST -j '{"title": "foo", "body": "bar", "userId": 1}' https://jsonplaceholder.typicode.com/posts
Sending Query Parameters
$ httpx-cli -p userId=1 https://jsonplaceholder.typicode.com/posts
Specifying Custom Headers
$ httpx-cli -h "Authorization" "Bearer TOKEN" https://api.example.com/resource
Handling Cookies
$ httpx-cli --cookies "session_id" "12345" https://example.com
Following Redirects
$ httpx-cli --follow-redirects https://example.com
Disabling SSL Verification
$ httpx-cli --no-verify https://example.com
Downloading Files
$ httpx-cli --download image.jpg https://example.com/image.jpg
Verbose Output
$ httpx-cli -v https://example.com
Authentication
$ httpx-cli --auth username:password https://api.example.com/resource
Sending Form Data
$ httpx-cli -m POST -d "key1=value1&key2=value2" https://example.com/submit
Sending Files
$ httpx-cli -m POST -f "file1@/path/to/file1.txt" -f "file2@/path/to/file2.txt" https://example.com/upload
HTTP/2 Support
$ httpx-cli --http2 https://example.com
Certainly, here’s a writeup with examples for using the httpx
Python library:
httpx - the Python HTTP Client Library
This is essentially the same tool, but as library for use in your own Python code.
Installation
$ pip install httpx
Sending a GET Request
import httpx
async def send_get_request():
async with httpx.AsyncClient() as client:
response = await client.get("https://jsonplaceholder.typicode.com/posts/1")
print(response.text)
# Run the asynchronous function
import asyncio
asyncio.run(send_get_request())
This code sends a GET request to the specified URL and prints the response content.
Sending a POST Request with JSON Data
import httpx
async def send_post_request():
async with httpx.AsyncClient() as client:
data = {"title": "foo", "body": "bar", "userId": 1}
response = await client.post("https://jsonplaceholder.typicode.com/posts", json=data)
print(response.text)
# Run the asynchronous function
import asyncio
asyncio.run(send_post_request())
Here, we send a POST request with JSON data to create a new post.
Sending Query Parameters
import httpx
async def send_request_with_query_params():
async with httpx.AsyncClient() as client:
params = {"userId": 1}
response = await client.get("https://jsonplaceholder.typicode.com/posts", params=params)
print(response.text)
# Run the asynchronous function
import asyncio
asyncio.run(send_request_with_query_params())
You can include query parameters in the request URL.
Specifying Custom Headers
import httpx
async def send_request_with_custom_headers():
async with httpx.AsyncClient() as client:
headers = {"Authorization": "Bearer TOKEN"}
response = await client.get("https://api.example.com/resource", headers=headers)
print(response.text)
# Run the asynchronous function
import asyncio
asyncio.run(send_request_with_custom_headers())
Use custom HTTP headers in the request by specifying them in the headers dictionary.
Handling Cookies
import httpx
async def send_request_with_cookies():
async with httpx.AsyncClient() as client:
cookies = {"session_id": "12345"}
response = await client.get("https://example.com", cookies=cookies)
print(response.text)
# Run the asynchronous function
import asyncio
asyncio.run(send_request_with_cookies())
You can send cookies with your request by providing a cookies dictionary.
Following Redirects
import httpx
async def send_request_with_redirects():
async with httpx.AsyncClient() as client:
response = await client.get("https://example.com", follow_redirects=True)
print(response.url)
# Run the asynchronous function
import asyncio
asyncio.run(send_request_with_redirects())
Set follow_redirects=True
to automatically follow HTTP redirects.
Disabling SSL Verification
import httpx
async def send_request_with_no_ssl_verify():
async with httpx.AsyncClient(verify=False) as client:
response = await client.get("https://example.com")
print(response.text)
# Run the asynchronous function
import asyncio
asyncio.run(send_request_with_no_ssl_verify())
Disable SSL verification by passing verify=False
when creating the client.